Hi!
Is there any way to pass an f-string into Lookup, to do something like the following?
visible=IsEqual(Lookup(f"{section_name}.options"),"Custom")
Lookup
is working properly if I replace the f-string with a pure string but it is not working this way.
Thanks!
Hi Andres,
Welcome to the community! And thanks for posting your question.
Iβve taken your case, and built a small app to check your case. In my case, it does seem to work. Here is my snippet:
from viktor import ViktorController
from viktor.parametrization import ViktorParametrization, NumberField, OptionField, Section, IsEqual, Lookup
section_name = 'section'
visible = IsEqual(Lookup(f"{section_name}.options"), "Custom")
class Parametrization(ViktorParametrization):
section = Section('My section')
section.options = OptionField('My options', ['Option 1', 'Option 2', 'Custom'])
section.custom_field = NumberField('Custom field', visible=visible)
class Controller(ViktorController):
label = '...'
parametrization = Parametrization
And here is a GIF that shows the result:
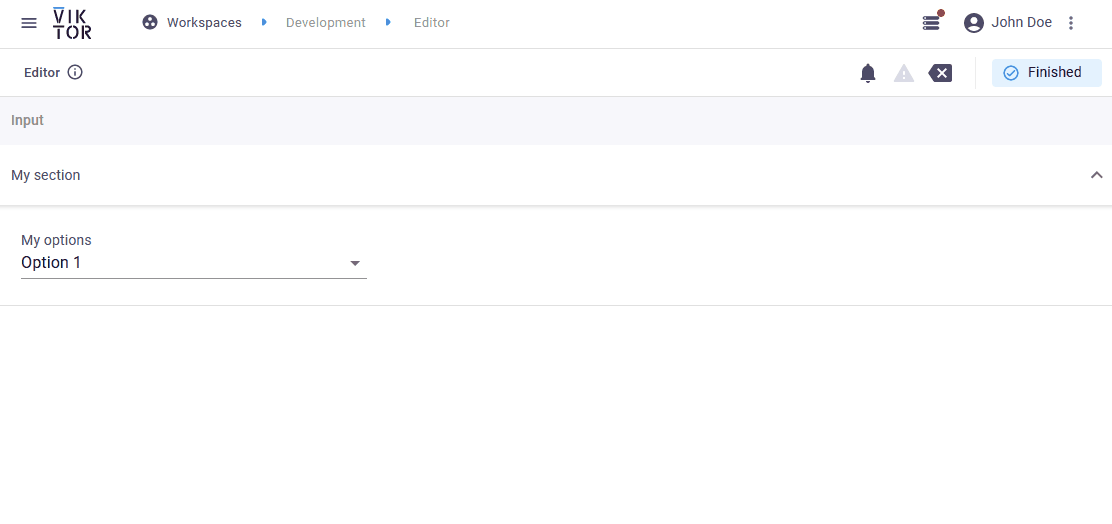
Could it be that the name that you have given the variable section_name
is misspelt? Iβm curious to hear if this helps to solve your issue.
Hi Marcel,
Thanks for your reply. I want to do something like the block of code below:
- I want to have to sections, and the
NumberField
field should only appear if we Custom
is chosen, for each of the fields. With this script below this doesnβt seem to work. What may I be doing wrong?
Thanks!
from viktor import ViktorController
from viktor.parametrization import ViktorParametrization, NumberField, OptionField, Section, IsEqual, Lookup
def add_specs(section):
section.options = OptionField(
"Options",
options=["Default", "Custom"],
default="Default",
)
section.value = NumberField(
"Value",
min=0,
visible=IsEqual(Lookup(f"{section}.options"), "Custom"),
)
class Parametrization(ViktorParametrization):
section_1 = Section('My section')
add_specs(section_1)
section_2 = Section('My section 2')
add_specs(section_2)
class Controller(ViktorController):
label = '...'
parametrization = Parametrization```
Ah yes, now I understand. I really like your approach of avoiding repetitive tasks!
Your implementation, however, does not work, as the object that you are sending as argument in your function is a Section
object. You can check yourself what type of string is created if you add the object to that f-string statement. For example, if I print your f-string you created, I get the following:
"<viktor.parametrization.Section object at 0x0000022E99D7B4D0>.options"
To make your code work with minimal changes, you can do the following:
from viktor import ViktorController
from viktor.parametrization import ViktorParametrization, NumberField, OptionField, Section, IsEqual, Lookup
def add_specs(section: Section, section_label: str):
section.options = OptionField(
"Options",
options=["Default", "Custom"],
default="Default",
)
section.value = NumberField(
"Value",
min=0,
visible=IsEqual(Lookup(f"{section_label}.options"), "Custom"),
)
class Parametrization(ViktorParametrization):
section_1 = Section('My section')
add_specs(section_1, 'section_1')
section_2 = Section('My section 2')
add_specs(section_2, 'section_2')
class Controller(ViktorController):
label = '...'
parametrization = Parametrization
That was helpful, thanks for showing me this approach!
1 Like